Context APIs
APIs to fetch locally stored context on a user device
Real-time Smart Activity Recognition
Context360 leverages fusion of mobile sensors and passive location monitoring to recognize and infer user physical activities as they happen. Each activity field has a start and end time and activity type. The following list shows currently supported activities. The list is periodically updated as more features are developed.
List of Activities (in 3.0.1)
Arrived at HOME
Left HOME
Arrived at WORK
Left WORK
Lunch break
Wake up in the morning
Sleep
Smart Activity history APIs
To get a list of smart activities between start and end date use the below API
- (NSArray <C360SmartActivityContext *> *) fetchSmartActivityHistoryFrom:(NSDate *) start Through:(NSDate *) end;
func fetchSmartActivityHistoryFrom(start: NSDate, Through end: NSDate) -> Array<C360SmartActivityContext> {
}
Fetch smart activities going back "n" seconds in the past
Allows an app to fetch smart activities which have a start date of "n" seconds in the past.
- (NSArray <C360SmartActivityContext *> *) fetchSmartActivityHistoryForThePast:(NSTimeInterval) seconds;
func fetchSmartActivityHistoryForThePast(seconds: NSTimeInterval) -> Array<C360SmartActivityContext> {
}
C360 Headers
Look under Senselytics Framework headers if you need more information about objects returned by APIs. Headers are well documented. Feel free to contact developer support should the need to get more information arise.
Besides passively collecting user's context and pushing this data to the Context360 cloud, Senselytics framework also allows a client app to directly fetch user's context stored on the device. Senselytics maintains a history of user's location, motion and fencing activities to enable high level of application personalization. This data can also be used by local AI or bots allowing them to gain a new level of intelligence about the user. This section provides details on the APIs provided by the framework.
User Interest Profiles
If location monitoring is enabled from Context360 dashboard, the framework profiles users based upon user's significant location visits. Application can access the list of profiles created by the framework by using the following API. Note that profiles are returned based upon location visits between the start and end date passed to the API.
List of User Interests available in framework v2.6.7
FUTURE TRAVELLERS
BUSINESS PROFESSIONALS,
REAL ESTATE FOLLOWERS,
BOOKWORMS,
ENTERTAINMENT ENTHUSIASTS,
MOVIE LOVERS,
MUSIC LOVERS,
SLOTS PLAYERS,
TECH NEWS,
SCIENTIFIC NEWS,
AUTO ENTHUSIASTS,
ACTIVE AUTO BUYERS,
FASHIONISTAS,
DINING LOVERS,
HEALTH & FITNESS ENTHUSIASTS,
HOME & GARDEN PROS,
LGBT,
NEW MOTHERS,
PARENTING & EDUCATION,
PET OWNERS,VALUE SHOPPERS,
PROFESSIONAL SPORTS FANS,
- (void) fetchUserInterestsFrom:(NSDate *) start Through:(NSDate *) end withCompletionHandler:(C360UserInterestCompletionHandler) handler;
func fetchUserInterests(from start: Date, through end: Date, with handler: C360UserInterestCompletionHandler) {
}
Trips monitoring
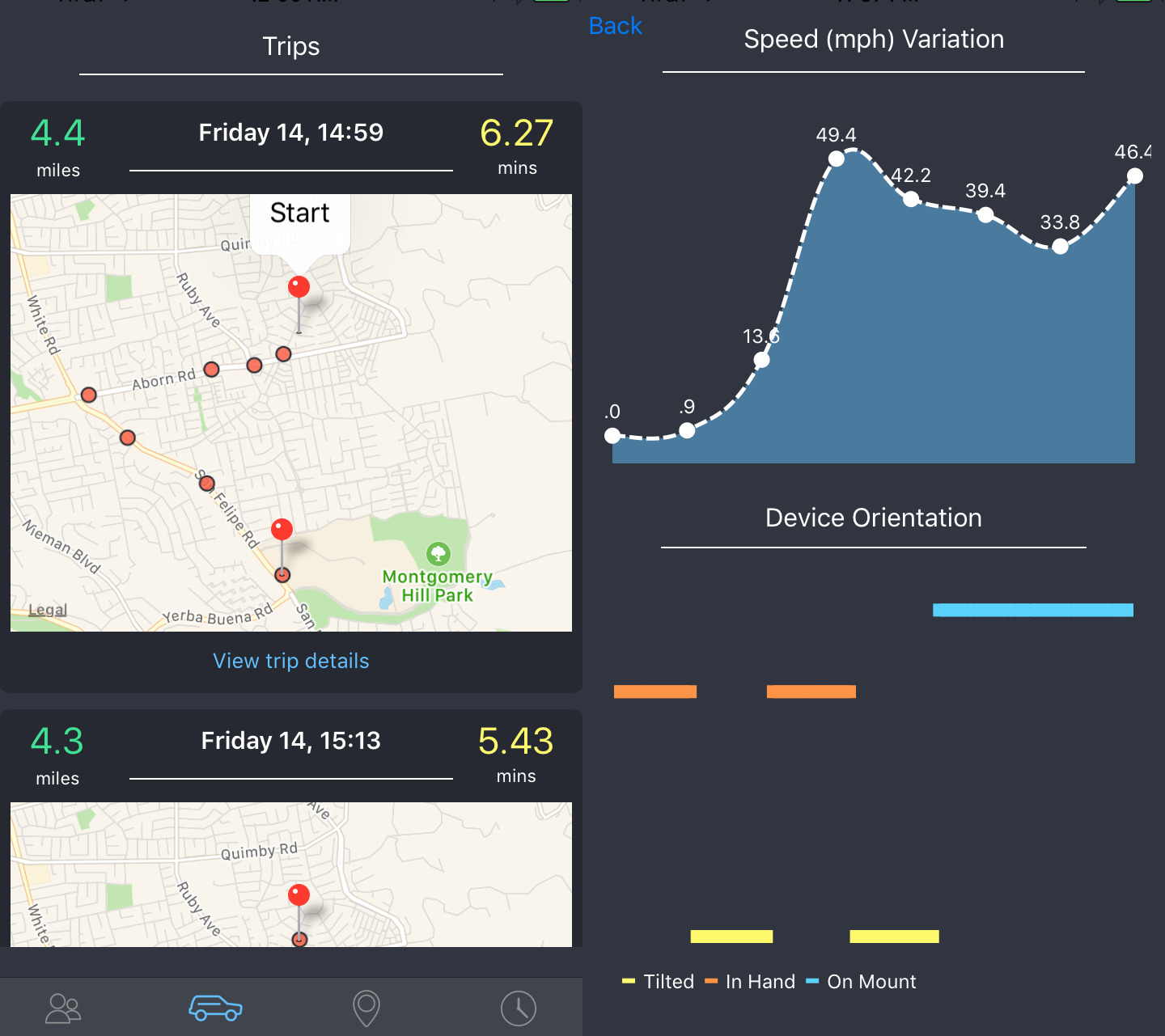
If trips monitoring is enabled from Context360 dashboard, the framework automatically detects user's motion and fuses it with location visits to record user's driving history. This data can be used to offer suggestions of local businesses on user way to home or work. Trips are returned as C360TripContext objects which contains an array of waypoints. You can use MapKit framework to draw routes or just find the distance of the trip.
- (NSArray <C360TripContext *> *) fetchTripHistoryFrom:(NSDate *) start Through:(NSDate *) end;
func fetchTripHistory(from start: Date, through end: Date) -> [C360TripContext] {
}
Reverse-geocoding using Senselytics smart geocoding
Senselytics allows you to reverse geocode a GPS coordinate into location name/category. This API also benefits from all the learning data gathered by the framework on the device to improve the geocoding results in case of ambiguity. For example a shopping mall can have a lot of nearby locations show up when performing geocoding and choosing the nearest entry is not always right. Use the "learn" flag to return results based on smart geocoding functionality in the framework.
Note
When "learn" flag is false , results are ordered in the increasing order of nearness to the coordinate.
- (void) fetchGeocodedLocationsFor:(C360LocationContext *) locationCtx useLearning:(BOOL)learn withCompletionHandler:(C360GeocodingCompletionHandler) handler;
C360ContextEngine.shared().fetchGeocodedLocations(for: , useLearning: , withCompletionHandler: ([C360GeocodedLocationContext]?) -> Void#>)
Location history APIs
If location monitoring is enabled from Context360 dashboard, the framework saves a history of significant location visits. Below APIs allow an app to request for user's location visits starting from a date in the past.
Fetch ongoing location context
Allows an app to fetch a location activity which is ongoing. Note that "end" field of the C360LocationContext object returned by this API will be nil.
- (C360LocationContext *) fetchLocationActivityOngoing;
func fetchLocationActivityOngoing() -> C360LocationContext {
}
Fetch location activities going back "n" seconds in the past
Allows an app to fetch location activities which have a start date of "n" seconds in the past.
- (NSArray <C360LocationContext *> *) fetchLocationActivityHistoryForThePast:(NSTimeInterval) seconds;
func fetchLocationActivityHistoryForThePast(seconds: NSTimeInterval) -> Array<C360LocationContext> {
}
Fetch location activities between two dates in the past
Allows an app to fetch location activities which have a start dates between the provided start and end dates in the past.
- (NSArray <C360LocationContext *> *) fetchLocationActivityHistoryFrom:(NSDate *) start Through:(NSDate *) end;
func fetchLocationActivityHistoryFrom(start: NSDate, Through end: NSDate) -> Array<C360LocationContext> {
}
Motion history APIs
If motion monitoring is enabled from Context360 dashboard, the framework saves a history of motion activities as the smartphone moves. Below APIs allow an app to request for user's motion activities starting from a date in the past.
Along with user motion, the device orientation context is also saved. This information can be used to set rules from Senselytics dashboard. For example if the user is "stationary" and device is "in hand". Here is the table which describes possible states for user motion activities and device orientations.
Note that a few states in the table below are undetectable due to the assumption that user must have physical contact with the device for the framework to be able to predict the posture.
On Phone Call | In Hand | Tilted | In Pocket | In Arm Band | Flat Face Up | Flat Face Down | On Mount | |
---|---|---|---|---|---|---|---|---|
Any (Unknown) | YES | YES | YES | YES | YES | YES | YES | YES |
Stationary | YES | YES | - | YES | - | - | - | - |
Reclining | - | YES | - | - | - | - | - | - |
Walking | YES | YES | - | YES | YES | - | - | - |
Running | - | - | - | YES | YES | - | - | - |
Driving | YES | YES | YES | YES | - | YES | YES | YES |
Flying | YES | YES | YES | YES | - | YES | YES | YES |
Fetch ongoing motion context
Allows an app to fetch a motion activity which is ongoing. Note that "end" field of the C360MotionContext object returned by this API will be nil.
- (C360MotionContext *) fetchMotionActivityOngoing;
func fetchMotionActivityOngoing() -> C360MotionContext {}
Fetch motion activities going back "n" seconds in the past
Allows an app to fetch motion activities which have a start date of "n" seconds in the past.
- (NSArray <C360MotionContext *> *) fetchMotionActivityHistoryForThePast:(NSTimeInterval) seconds;
func fetchMotionActivityHistoryForThePast(seconds: NSTimeInterval) -> Array<C360MotionContext> {
}
Fetch motion activities between two dates in the past
Allows an app to fetch motion activities which have a start dates between the provided start and end dates in the past.
- (NSArray <C360MotionContext *> *) fetchMotionActivityHistoryFrom:(NSDate *) start Through:(NSDate *) end;
func fetchMotionActivityHistoryFrom(start: NSDate, Through end: NSDate) -> Array<C360MotionContext> {
}
Geofence context APIs
Below APIs return a dictionary of arrays. Keys in the dictionary are the geofence identifiers (NSString). Each key stores an array of geofence context activities. If a user has not yet triggered any geofence , this API will still return the actively monitored geofence on the device. Please note that both the start and end fields of C360GeofenceContext can be nil. Apps can fetch various stats on geofences like walk-in, drive-by etc.
Fetch geofence activities going back "n" seconds in the past
Allows an app to fetch geofence trigger activities which have a start date of "n" seconds in the past.
- (NSDictionary<NSString *, NSArray<C360GeofenceContext *> *> *) fetchGeofenceActivityHistoryForThePast:(NSTimeInterval) seconds
Fetch geofence activities between two dates in the past
Allows an app to fetch geofence trigger activities which have a start dates between the provided start and end dates in the past.
- (NSDictionary<NSString *, NSArray<C360GeofenceContext *> *> *) fetchGeofenceActivityHistoryFrom:(NSDate *) start Through:(NSDate *) end;
Rule execution record APIs
When rules execute on a device, some metadata associated with the rule is stored locally. This API gives access to this information.
- (NSArray <C360RuleExecutionRecord *> *) fetchRuleExecutionHistoryFrom:(NSDate *) start Through:(NSDate *) end
func fetchRuleExecutionHistory(from start: Date, through end: Date) -> [C360RuleExecutionRecord] {
}
Updated over 7 years ago