Integration steps
Also look at our example apps repository
Check with Context360 team to get access to our example apps repository. This repo contains example apps which demonstrate the use of iOS SDK APIs and may also speed up your testing.
Getting user authorization on Location and Push permissions
Please note that SDK does not automatically trigger iOS permission dialogs for getting permissions on collecting location data from the end user, So It is the responsibility of your application to invoke calls to the iOS APIs to trigger opt-ins at the right time before calling SDK APIs.
Under capabilities in your Xcode project enable "Remote Notifications" add the following "Background Modes" capabilities. Note that enabling notifications based on iBeacons requires adding the bluetooth background mode capability. If you do not plan to enable iBeacon support then this mode can be skipped.
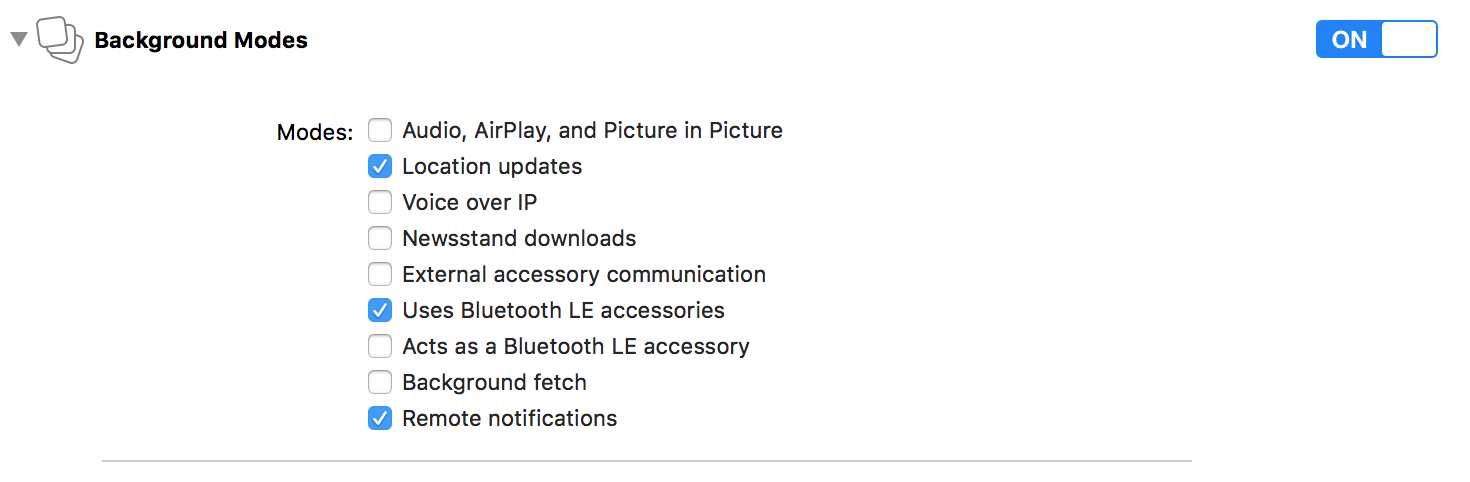
Open the Info.plist file as source code:
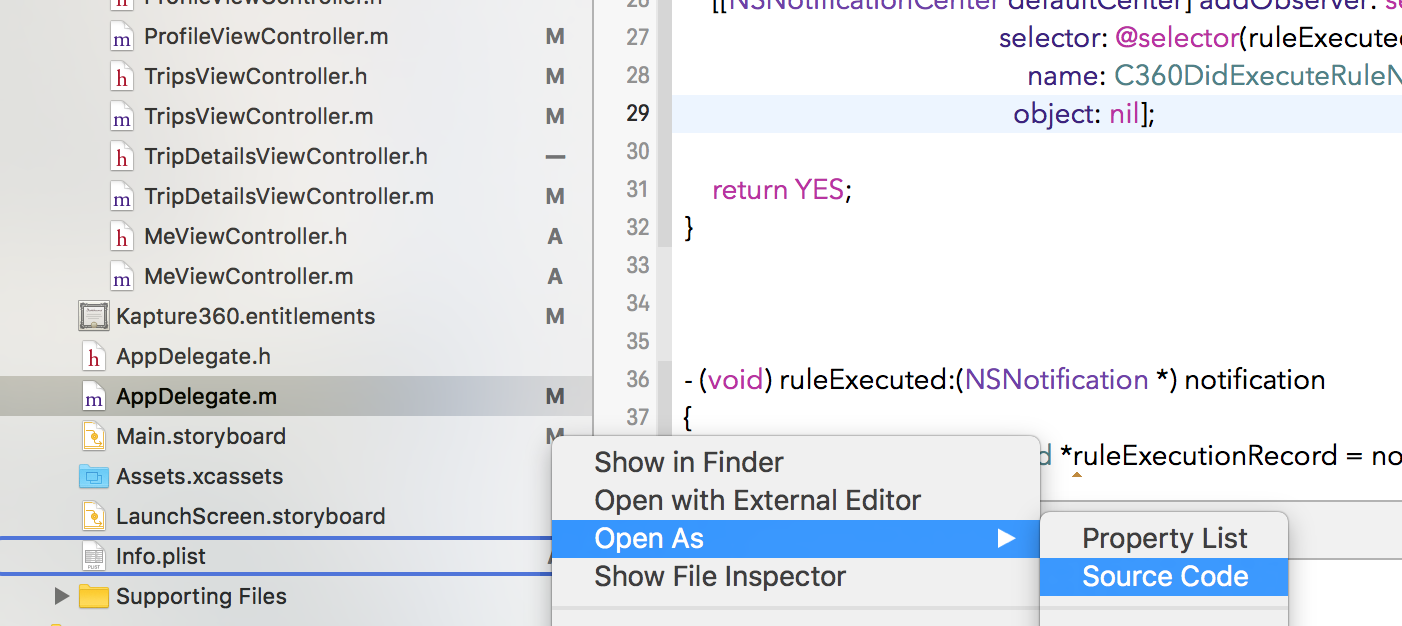
Add the following keys to your Info.plist file. Replace YOUR_API_KEY with the key you received after creating an app on the Senselytics Dashboard.
<key>Senselytics</key>
<dict>
<key>APIKey</key>
<string>YOUR_API_KEY</string>
</dict>
For On premise installation. You need to also add "OnPremServerBaseURL" key.
You need to add this key to the Info.plist file. Use the below source instead.
For on-premise install replace the YOUR_SERVER_BASE_URL with appropriate base url.
<key>Senselytics</key>
<dict>
<key>APIKey</key>
<string>YOUR_API_KEY</string>
<key>OnPremServerBaseURL</key>
<string>YOUR_SERVER_BASE_URL</string>
</dict>
Next set of steps require you to add some code to your application delegate file. (AppDelegate.m)
Add call to import the C360 header.
@import c360;
import c360
In function
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplicationLaunchOptionsKey: Any]?) -> Bool {
}
add call to the following Context360 function
[[C360ContextEngine sharedContextEngine] startEngineWithUserIdentifier:@"any_user_identifier"];
C360ContextEngine.shared().startEngine(withUserIdentifier: "any_user_identifier")
Note that the calls above signs up a user and starts the context engine. These calls should ideally be after the user logs into the application. Thats where you would have a valid username etc.
Override the function (add this function to your application delegate implementation file if it does not already exist)
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken: (NSData *)deviceToken
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
}
and place a call to register the device with C360 cloud within the below function
[[C360ContextEngine sharedContextEngine] registerRemoteNotificationToken:deviceToken];
C360ContextEngine.shared().registerRemoteNotificationToken(deviceToken)
Override the function
- (void) application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable: Any], fetchCompletionHandler handler: @escaping (_: UIBackgroundFetchResult) -> Void) {
}
and place a call to C360 function to handle remote notifications. Note that the example shows function's completion handler calling iOS fetch data status updater. It is not mandatory to call it from this function but since this function does asynchronous task in the background calling OS handler function with fetch result outside this handler may result in iOS not giving enough CPU cycles to complete the background task.
[[C360ContextEngine sharedContextEngine] handleRemoteNotification:userInfo
withCompletionHandler:^{
completionHandler(UIBackgroundFetchResultNewData);
}];
C360ContextEngine.shared().handleRemoteNotification(userInfo, withCompletionHandler: {() -> Void in
handler(.newData)
})
Run the application in Xcode. Although the SDK supports running on the simulator build we recommend to test on an actual device. You should see this in your log prints if the integration was successful.
IntegrationTest[5192:1246456] [C360 Senselytics] Version 3.1.0
Voila! You have successfully integrated the iOS framework
Stopping framework services
Should there be a need to stop Senselytics framework's services from the host app, an API to request a stop on all services exists within the framework. To restart just call the startEngineWithApiKey . Note that Context360 Dashboard also provides a way to stop all services on specific segment of devices.
[[C360ContextEngine sharedContextEngine] stopEngine];
C360ContextEngine.shared().stopEngine()
Updated over 7 years ago